Problem
There are n kids with candies. You are given an integer array candies, where each candies[i] represents the number of candies the ith kid has, and an integer extraCandies, denoting the number of extra candies that you have. Return a boolean array result of length n, where result[i] is true if, after giving the ith kid all the extraCandies, they will have the greatest number of candies among all the kids, or false otherwise. Note that multiple kids can have the greatest number of candies. Example 1: Input: candies = [2,3,5,1,3], extraCandies = 3 Output: [true,true,true,false,true] Explanation: If you give all extraCandies to: - Kid 1, they will have 2 + 3 = 5 candies, which is the greatest among the kids. - Kid 2, they will have 3 + 3 = 6 candies, which is the greatest among the kids. - Kid 3, they will have 5 + 3 = 8 candies, which is the greatest among the kids. - Kid 4, they will have 1 + 3 = 4 candies, which is not the greatest among the kids. - Kid 5, they will have 3 + 3 = 6 candies, which is the greatest among the kids. Example 2: Input: candies = [4,2,1,1,2], extraCandies = 1 Output: [true,false,false,false,false] Explanation: There is only 1 extra candy. Kid 1 will always have the greatest number of candies, even if a different kid is given the extra candy. Example 3: Input: candies = [12,1,12], extraCandies = 10 Output: [true,false,true] Constraints:
|
Solution
class Solution: def kidsWithCandies(self, candies: List[int], extraCandies: int) -> List[bool]: ans=[] for i in range(len(candies)): if candies[i]+extraCandies >= max(candies): ans.append(True) else: ans.append(False) return ans |
이번 문제를 풀고, 복습하는 시간을 가져야겠다.
이번문제는 아이들 사탕 나눠주는 문제이다. 스토리텔링을 한 문제였다.
이 문제를 풀면서 사탕이 싫어졌다....
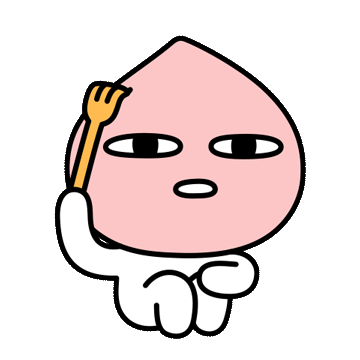
어째든 첫 번째로, 새로운 배열 ans를 설정해 주었다. 그리고 for문을 선언해주었는데 조건 candies의 길이로 정했다.
왜냐하면 candies 배열을 각각 비교해야 하기 떄문이죵
그리고 for문 안에 if문을 설정해줘야 합니다. Explanation의 예제를 보면 각 배열의 숫자와 extraCandies 합이 Candies 배열 중 가장 큰 숫자와 비교해서 True or false로 나뉜다.
if문을 풀어보면
candies[0]+extraCandies 2 + 3 = 5 > 5 = True
candies[0]+extraCandies 3 + 3 = 6 > 6 = True
candies[0]+extraCandies 5 + 3 = 8 > 5 = True
candies[0]+extraCandies 1 + 3 = 4 > 5 = false
candies[0]+extraCandies 3 + 3 = 6 > 5 = True
요런식으로 풀이 된다.
그리고 다시 ans.append를 사용해 ans배열에 추가한다.
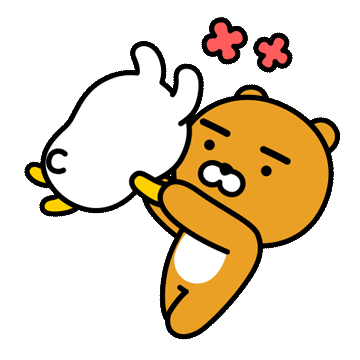
Output: [true,true,true,false,true]
'초보코딩' 카테고리의 다른 글
Leetcode - 1365. How Many Numbers Are Smaller Than the Current Number (0) | 2021.08.18 |
---|---|
LeetCode - 771. Jewels and Stones (0) | 2021.08.14 |
LeetCode - 1470. Shuffle the Array (0) | 2021.08.08 |
LeetCode - 1672. Richest Customer Wealth (0) | 2021.08.06 |
LeetCode - 1480. Running Sum of 1d Array (0) | 2021.08.05 |